Last Updated September 25th, 2019
How to add Featured Image Thumbnail Preview to WordPress Posts Column.
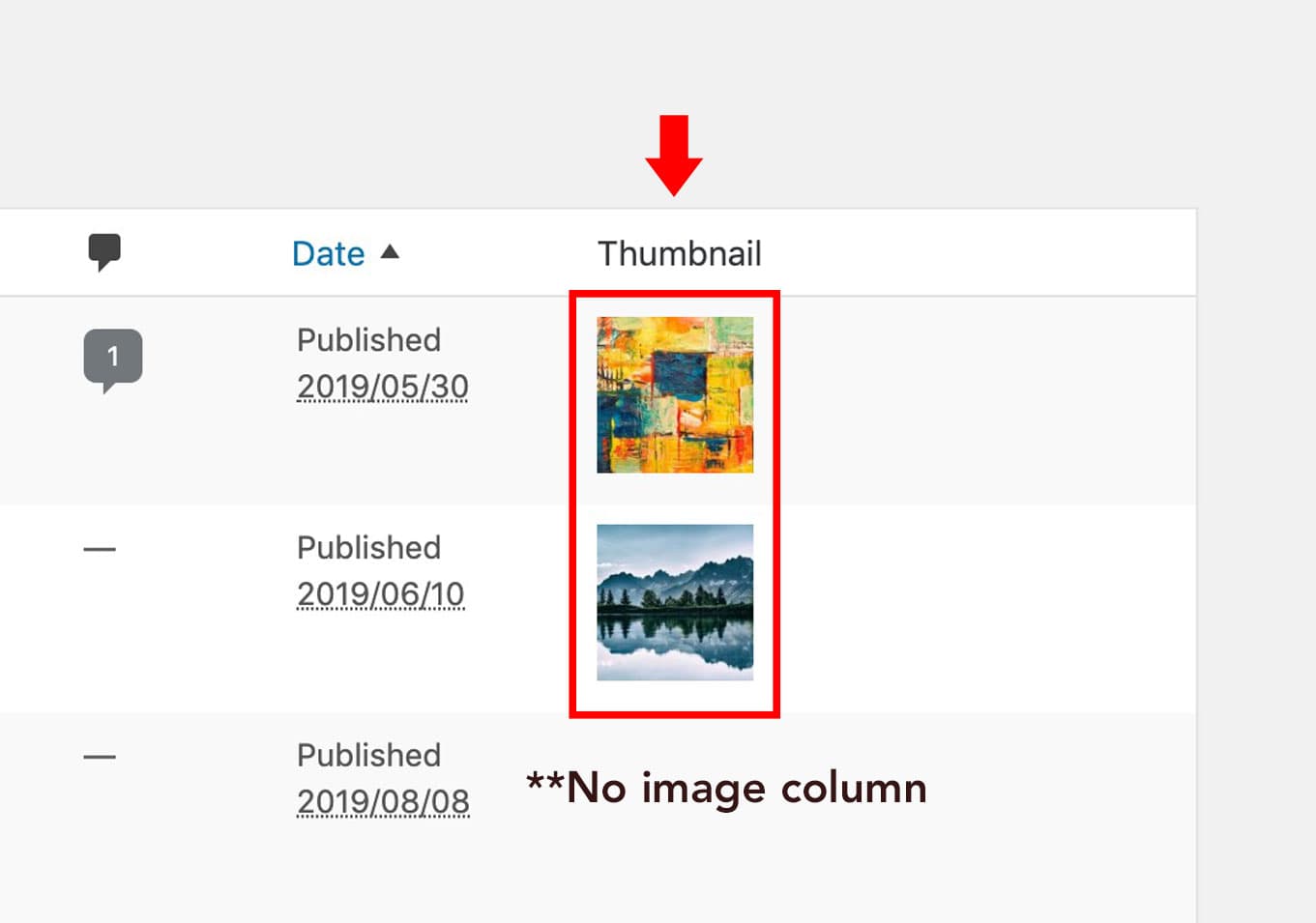
In this short tutorial, I will show you how to easily it add featured image thumbnail preview to WordPress posts on the admin side.
This can come in handy when you’re working on a WordPress plugin or custom post type and will like to show additional information to the user when on the WordPress admin page.
As you can see from the image below, the default WordPress posts list shows, title, author, categories, tags, comment count and date.
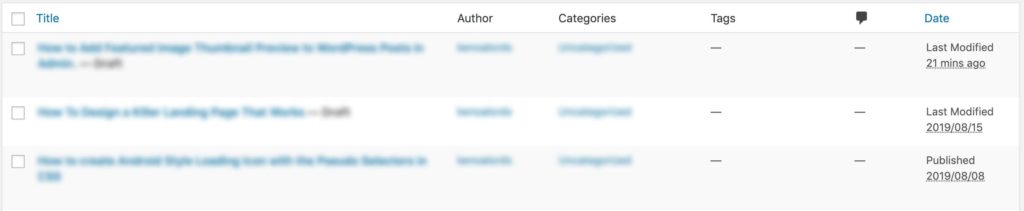
We will now add the featured image thumbnail preview using WordPress actions and filters.
WordPress ships with filters and actions that can be used to alter its core without editing the WordPress core.
We will be using manage_{$post_type}_posts_columns and manage_{$post_type}_posts_custom_column to alter the posts column and add a thumbnail preview.
The Solution
First, open your functions.php file within your active theme with any code editor of your choice.
Then input the following at the end of the file
# functions.php
add_filter( 'manage_posts_columns', 'manage_posts_columns_add_thumbnail', 10, 1 );
function manage_posts_columns_add_thumbnail($columns){
$columns['thumbnail'] = __('Thumbnail', 'theme_text_domain');
return $columns;
}
As you can see, the code above hooks into the manage_posts_columns filter and tells WordPress to add a new column “Thumbnail“.
Now here comes the fun part, displaying the thumbnail preview.
# functions.php
add_action( 'manage_posts_custom_column', 'posts_custom_columns', 10, 2 );
function posts_custom_columns( $column, $post_id ){
switch ( $column ){
case "thumbnail":
$thumb = get_the_post_thumbnail_url( $post_id, 'thumbnail' );
echo ($thumb) ? '<img src="'.$thumb.'" width="64" height="64">' : '';
break;
}
}
So let me explain what is going on here;
- First, we use the add_action WordPress hook to hook into the manage_posts_custom_column and provide a callback function.
- The callback function accepts 2 arguments, the column about been rendered and the ID of the post.
- By using PHP switch() statement, we check to see if the column is our custom column “thumbnail”.
- If it is, we use get_the_post_thumbnail_url() to grab the image link and store it in the variable $image.
- We then use the PHP ternary operator to check if a featured image exists in the current post.
- If it does, we display the image using <img /> else we return an empty string so that we don’t show a broken image icon.
If you don’t know what WordPress action hooks are, please check out the official docs.
Complete Code
# functions.php
// Add the thumbnail column
add_filter( 'manage_posts_columns', 'manage_posts_columns_add_thumbnail', 10, 1 );
function manage_posts_columns_add_thumbnail($columns){
$columns['thumbnail'] = __('Thumbnail', 'theme_text_domain');
return $columns;
}
// Display the thumbnail preview if available
add_action( 'manage_posts_custom_column', 'posts_custom_columns', 10, 2 );
function posts_custom_columns( $column, $post_id ){
switch ( $column ){
case "thumbnail":
$thumb = get_the_post_thumbnail_url( $post_id, 'thumbnail' );
echo ($thumb) ? '<img src="'.$thumb.'" width="64" height="64">' : '';
break;
}
}
Here’s what our final result looks like;
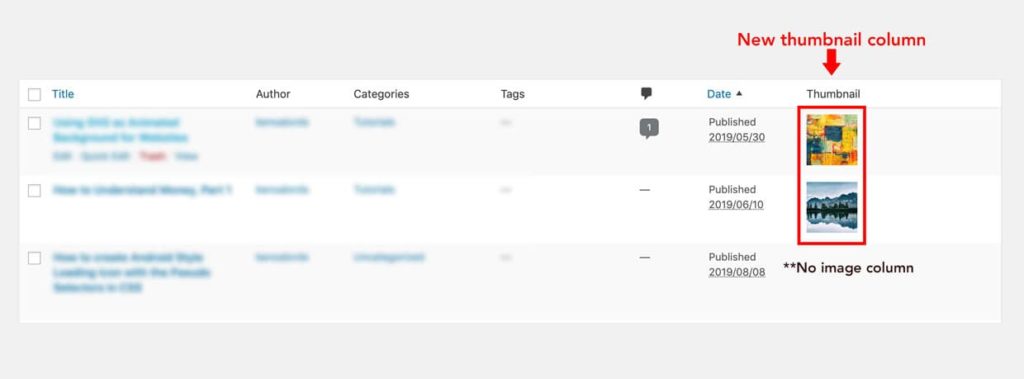
As you can see, the featured image thumbnail is now been displayed alongside its associated post.
Please note that you can add multiple columns like so;
add_filter( 'manage_posts_columns', 'manage_posts_columns_add_columns', 10, 1 );
function manage_posts_columns_add_columns($columns){
$columns['thumbnail'] = __('Thumbnail', 'theme_text_domain');
$columns['sticky'] = __('Sticky', 'theme_text_domain');
return $columns;
}
Then in your action callback using the switch statement, you can checck for multiple columns like so;
function my_posts_custom_columns( $column, $post_id ){
switch ( $column ){
case "thumbnail":
// Thumbnail operation goes here
break;
case "sticky":
// Sticky operation goes here
break;
}
}
I hope you found this useful.
Have questions? Ask them in the comment section below.
Joel D Canfield
July 8, 2020Excellent! Worked exactly as expected. I love not depending on plugins for my custom themes.