Last Updated September 14th, 2019
How to create an Android Style Loading Indicator with CSS
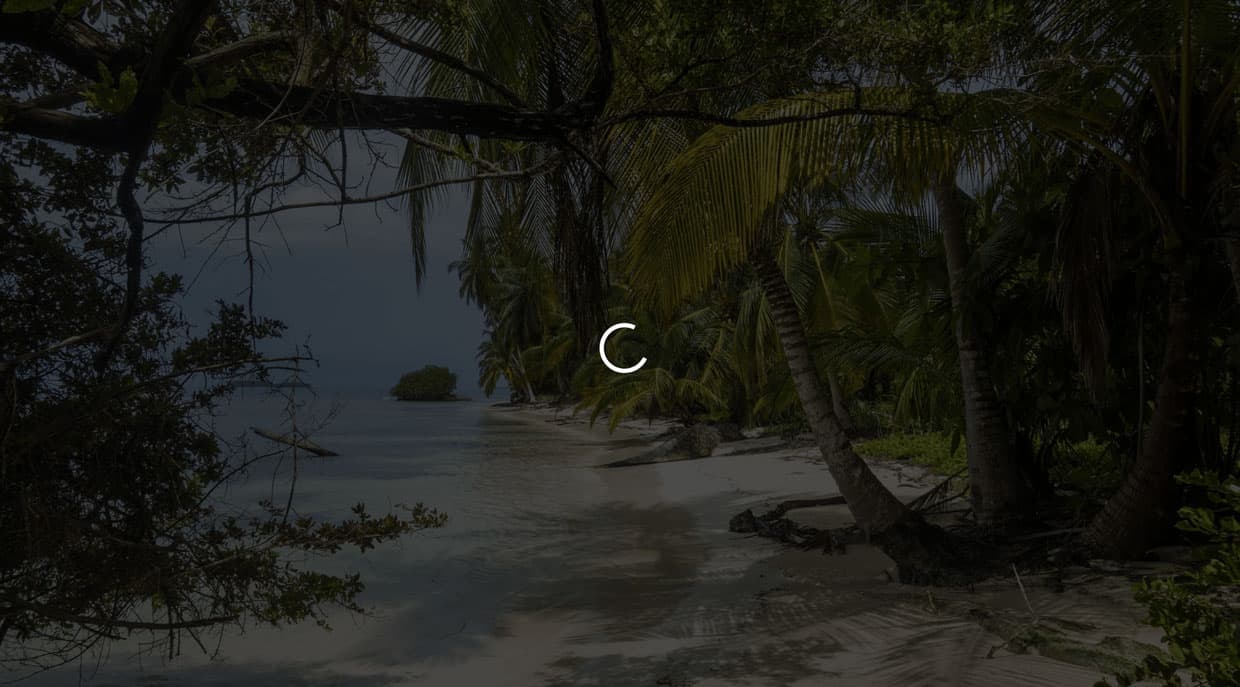
The Android loading indicator as seen on mobile applications is one of the simplest (in its design) and most recognized loading indicators in UI designs.
With lots of loading icon styles to choose from (check out https://loading.io), I still find the Android loading style more appealing and complementary in my designs.
Besides, using only CSS ensures that your website doesn’t make extra HTTP requests for loading gifs which is a good thing.
In this tutorial, I’d be showing you how easy it is to implement an Android loading icon or indicator in your website projects using only the :before and :after pseudo-elements and CSS keyframe animation.
We will only require two files for this demo just to keep things very simple. With your favourite code editor, create two files namely index.html and main.css then follow along.
#index.html
<html>
<head>
<meta charset="UTF-8">
<title>Android Loading Icon</title>
<link rel="stylesheet" href="main.css">
</head>
<body>
<div class="wrapper">
...
</div>
</body>
</html>
This forms the basic structure of our index.html file.
Now moving on the fun part of this tutorial, open your main.css file and copy and paste the code below.
/* Let's take care of some quick browser style resets */
html, body{
padding: 0;
margin: 0;
}
/* Now let's style our main wrapper element and give it a background image to make it look pretty */
.wrapper{
position: relative;
width: 100vw;
height: 100vh;
background: url('images/panama-4388225_1920.jpg') no-repeat center;
background-size: cover;
}
/*
* Let's activate the loading icon with an optional class e.g .is-loading
* This makes it easy to toggle with JavaScript
*/
/*
* First, let's create a simple spinning animation using CSS Keyframes
*/
@keyframes spinning{
0%{
transfrom: rotate(0deg);
}
100%{
transform: rotate(360deg);
}
}
/*
* Let's make the :before psuedo selector act as an overlay
* over any element it's applied to
*/
.is-loading:before{
content: "";
display: block;
background: rgba(0,0,0,.7);
position: absolute;
top: 0;
bottom: 0;
right: 0;
left: 0;
z-index: 2;
}
/*
* The :after psuedo selector will act as the spinning icon
* over the :before psuedo selector
*/
.is-loading:after{
content: "";
display: block;
position: absolute;
width: 48px;
height: 48px;
border-radius: 48px;
border: 5px solid #fff;
border-left-color: transparent;
top: 50%;
left: 50%;
margin-left: -24px;
margin-top: -24px;
z-index: 3;
animation: 1s spinning infinite linear; /* This set the animation property to make it spin */
}
We can now add the .is-loading class to the .wrapper div or any element of our choice to indicate a loading activity.
You can also customize the loading icon by fiddling with the CSS properties such as the size, border width, color and animation speed.
Hope you found this helpful? If you have any questions, please feel free to ask in the comment section below.